In recent years, we’ve seen Collaboration penetrate a lot of digital workflows and use-cases across many professions. Just within the Design and Software Engineering community, we see designers collaborate on design artifacts using tools like Figma, teams doing Sprint and Project Planning using tools like Mural and interviews being conducted using CoderPad. All these tools are constantly aiming to bridge the gap between an online and a physical world experience of executing these workflows and making the collaboration experience as rich and seamless as possible.
For the majority of the Collaboration Tools like these, the ability to share opinions with one another and have discussions about the same content is a must-have. A Commenting System that enables collaborators to annotate parts of a document and have conversations about them is at the heart of this concept. Along with building one for text in a WYSIWYG Editor, the article tries to engage the readers into how we try to weigh the pros and cons and attempt to find a balance between application complexity and user experience when it comes to building features for WYSIWYG Editors or Word Processors in general.
Representing Comments In Document Structure
In order to find a way to represent comments in a rich text document’s data structure, let’s look at a few scenarios under which comments could be created inside an editor.
- Comments created over text that has no styles on it (basic scenario);
- Comments created over text that may be bold/italic/underlined, and so on;
- Comments that overlap each other in some way (partial overlap where two comments share only a few words or fully-contained where one comment’s text is fully contained within text of another comment);
- Comments created over text inside a link (special because links are nodes themselves in our document structure);
- Comments that span multiple paragraphs (special because paragraphs are nodes in our document structure and comments are applied to text nodes which are paragraph’s children).
Looking at the above use-cases, it seems like comments in the way they can come up in a rich text document are very similar to character styles (bold, italics etc). They can overlap with each other, go over text in other types of nodes like links and even span multiple parent nodes like paragraphs.
For this reason, we use the same method to represent comments as we do for character styles, i.e. “Marks” (as they are so called in SlateJS terminology). Marks are just regular properties on nodes — speciality being that Slate’s API around marks (Editor.addMark
and Editor.removeMark
) handles changing of the node hierarchy as multiple marks get applied to the same range of text. This is extremely useful to us as we deal with a lot of different combinations of overlapping comments.
Comment Threads As Marks
Whenever a user selects a range of text and tries to insert a comment, technically, they’re starting a new comment thread for that text range. Because we would allow them to insert a comment and later replies to that comment, we treat this event as a new comment thread insertion in the document.
The way we represent comment threads as marks is that each comment thread is represented by a mark named as commentThread_threadID
where threadID
is a unique ID we assign to each comment thread. So, if the same range of text has two comment threads over it, it would have two properties set to the true
— commentThread_thread1
and commentThread_thread2
. This is where comment threads are very similar to character styles since if the same text was bold and italic, it would have both the properties set to true
— bold
and italic
.
Before we dive into actually setting this structure up, it’s worth looking at how the text nodes change as comment threads get applied to them. The way this works (as it does with any mark) is that when a mark property is being set on the selected text, Slate’s Editor.addMark API would split the text node(s) if needed such that in the resulting structure, text nodes are set up in a way that each text node has the exact same value of the mark.
To understand this better, take a look at the following three examples that show the before-and-after state of the text nodes once a comment thread is inserted on the selected text:
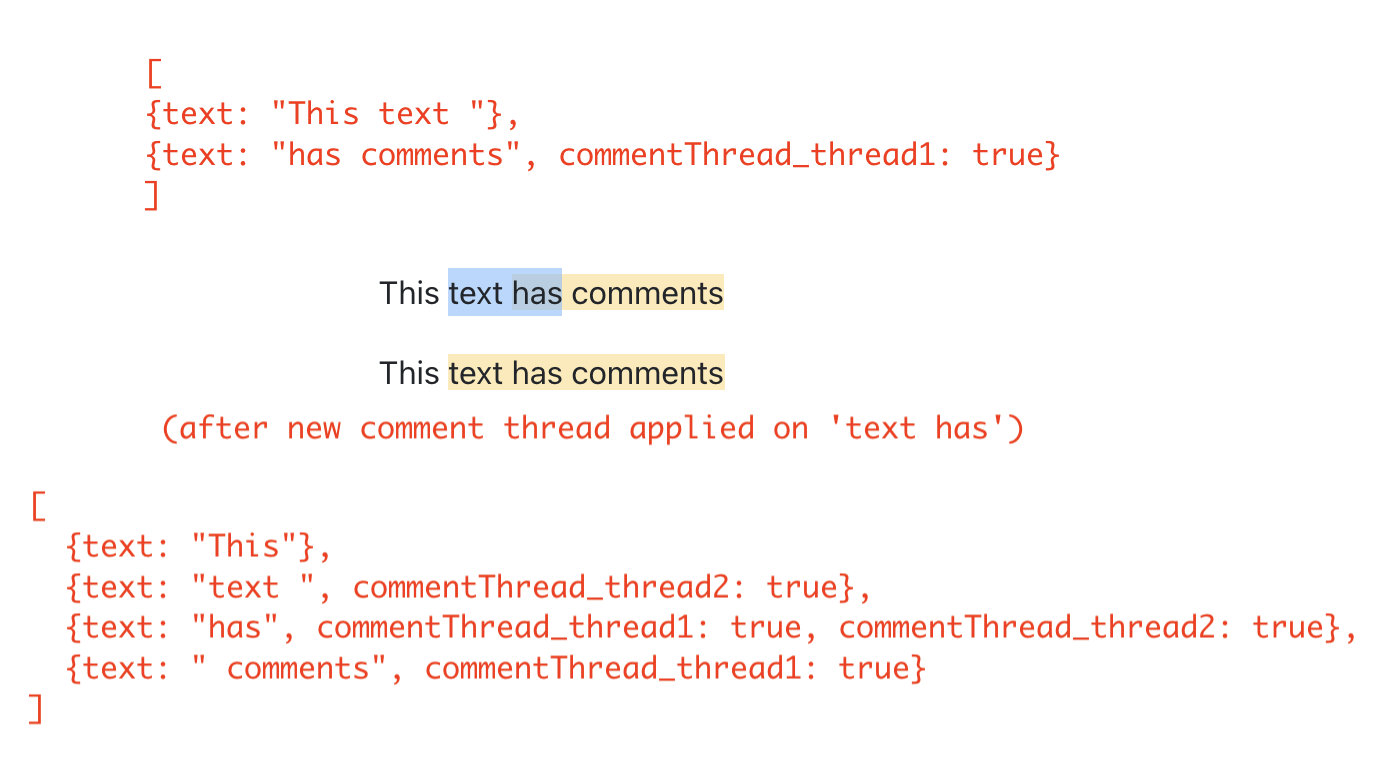
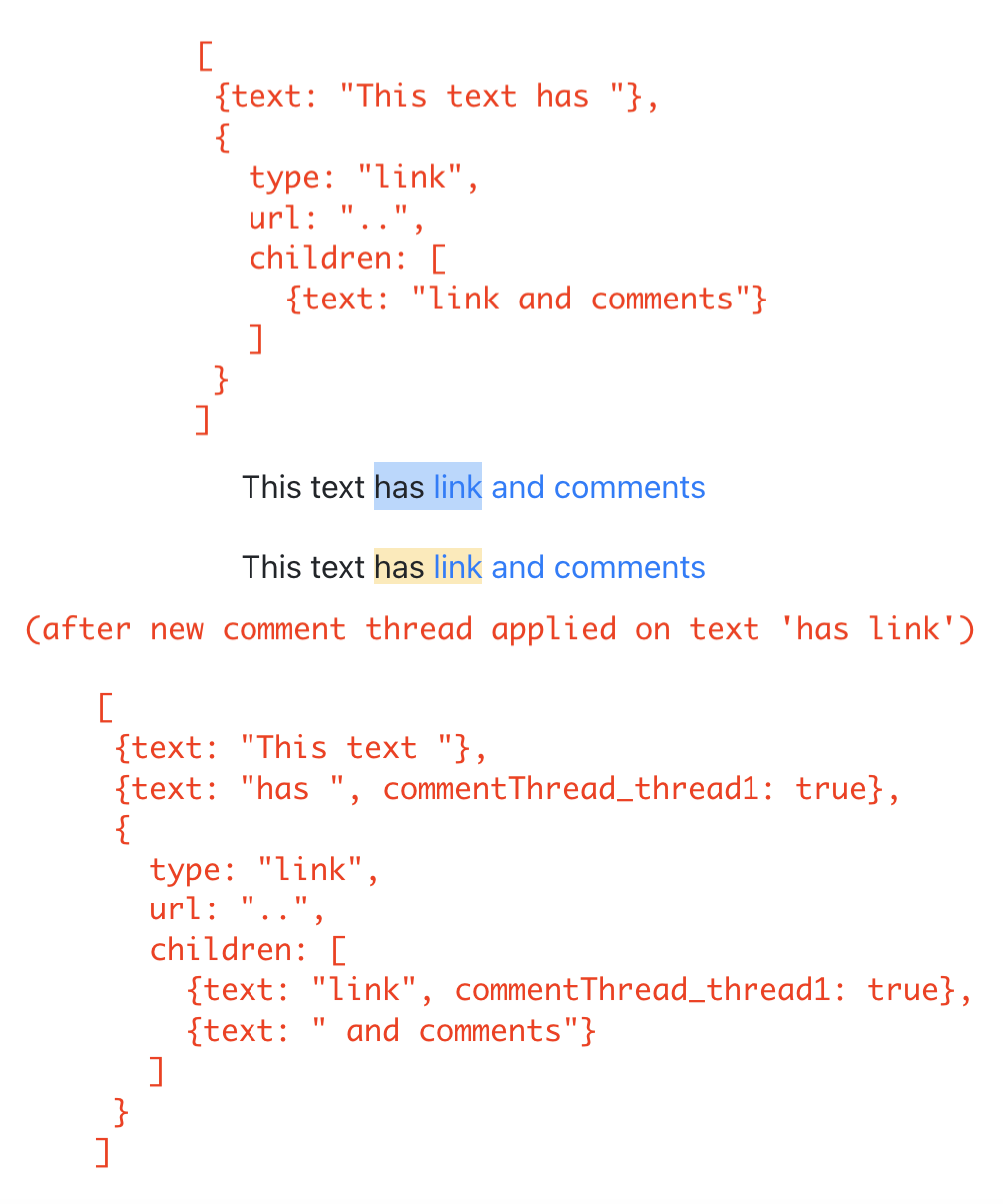
Highlighting Commented Text
Now that we know how we are going to represent comments in the document structure, let’s go ahead and add a few to the example document from the first article and configure the editor to actually show them as highlighted. Since we will have a lot of utility functions to deal with comments in this article, we create a EditorCommentUtils
module that will house all these utils. To start with, we create a function that creates a mark for a given comment thread ID. We then use that to insert a few comment threads in our ExampleDocument
.
# src/utils/EditorCommentUtils.js
const COMMENT_THREAD_PREFIX = "commentThread_";
export function getMarkForCommentThreadID(threadID) {
return `${COMMENT_THREAD_PREFIX}${threadID}`;
}
Below image underlines in red the ranges of text that we have as example comment threads added in the next code snippet. Note that the text ‘Richard McClintock’ has two comment threads that overlap each other. Specifically, this is a case of one comment thread being fully contained inside another.
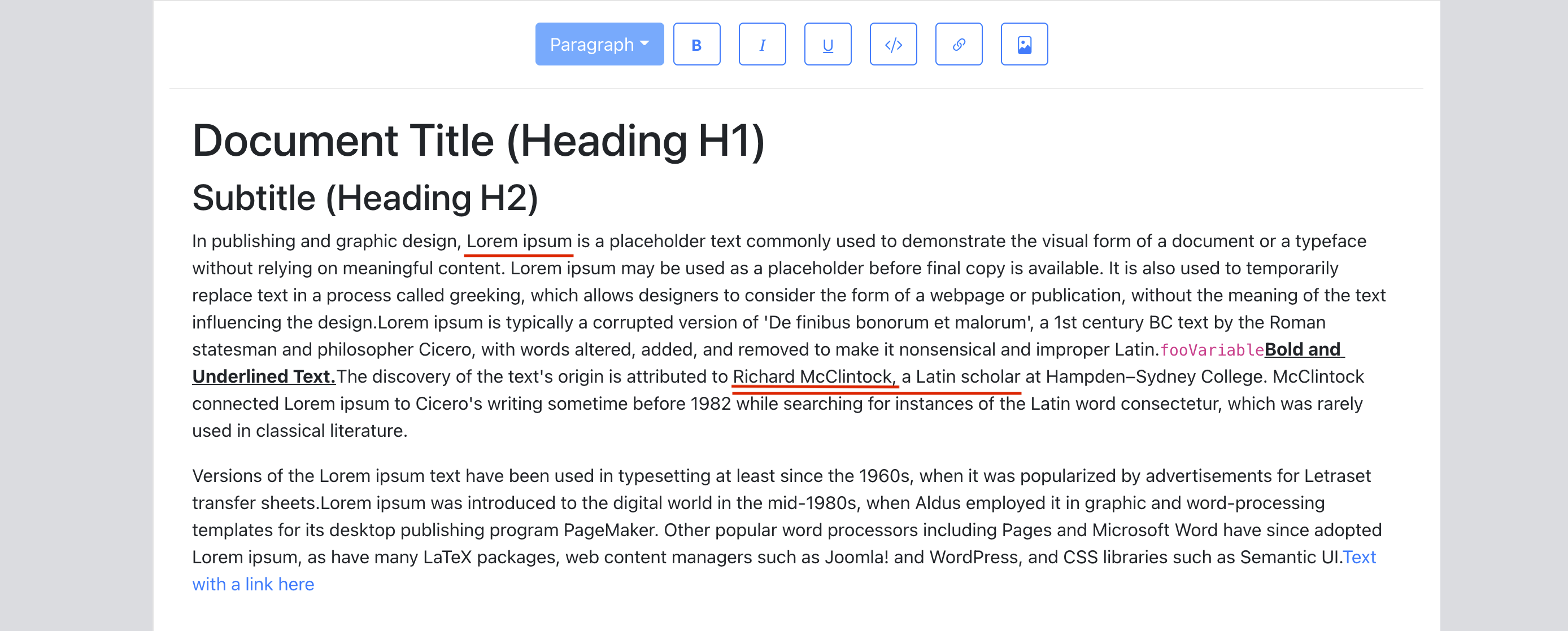
# src/utils/ExampleDocument.js
import { getMarkForCommentThreadID } from "../utils/EditorCommentUtils";
import { v4 as uuid } from "uuid";
const exampleOverlappingCommentThreadID = uuid();
const ExampleDocument = [
...
{
text: "Lorem ipsum",
[getMarkForCommentThreadID(uuid())]: true,
},
...
{
text: "Richard McClintock",
// note the two comment threads here.
[getMarkForCommentThreadID(uuid())]: true,
[getMarkForCommentThreadID(exampleOverlappingCommentThreadID)]: true,
},
{
text: ", a Latin scholar",
[getMarkForCommentThreadID(exampleOverlappingCommentThreadID)]: true,
},
...
];
We focus on the UI side of things of a Commenting System in this article so we assign them IDs in the example document directly using the npm package uuid. Very likely that in a production version of an editor, these IDs are created by a backend service.
We now focus on tweaking the editor to show these text nodes as highlighted. In order to do that, when rendering text nodes, we need a way to tell if it has comment threads on it. We add a util getCommentThreadsOnTextNode
for that. We build on the StyledText
component that we created in the first article to handle the case where it may be trying to render a text node with comments on. Since we have some more functionality coming that would be added to commented text nodes later, we create a component CommentedText
that renders the commented text. StyledText
will check if the text node it’s trying to render has any comments on it. If it does, it renders CommentedText
. It uses a util getCommentThreadsOnTextNode
to deduce that.
# src/utils/EditorCommentUtils.js
export function getCommentThreadsOnTextNode(textNode) {
return new Set(
// Because marks are just properties on nodes,
// we can simply use Object.keys() here.
Object.keys(textNode)
.filter(isCommentThreadIDMark)
.map(getCommentThreadIDFromMark)
);
}
export function getCommentThreadIDFromMark(mark) {
if (!isCommentThreadIDMark(mark)) {
throw new Error("Expected mark to be of a comment thread");
}
return mark.replace(COMMENT_THREAD_PREFIX, "");
}
function isCommentThreadIDMark(mayBeCommentThread) {
return mayBeCommentThread.indexOf(COMMENT_THREAD_PREFIX) === 0;
}
The first article built a component StyledText
that renders text nodes (handling character styles and so on). We extend that component to use the above util and render a CommentedText
component if the node has comments on it.
# src/components/StyledText.js
import { getCommentThreadsOnTextNode } from "../utils/EditorCommentUtils";
export default function StyledText({ attributes, children, leaf }) {
...
const commentThreads = getCommentThreadsOnTextNode(leaf);
if (commentThreads.size > 0) {
return (
<CommentedText
{...attributes}
// We use commentThreads and textNode props later in the article.
commentThreads={commentThreads}
textNode={leaf}
>
{children}
</CommentedText>
);
}
return <span {...attributes}>{children}</span>;
}
Below is the implementation of CommentedText
that renders the text node and attaches the CSS that shows it as highlighted.
# src/components/CommentedText.js
import "./CommentedText.css";
import classNames from "classnames";
export default function CommentedText(props) {
const { commentThreads, ...otherProps } = props;
return (
<span
{...otherProps}
className={classNames({
comment: true,
})}
>
{props.children}
</span>
);
}
# src/components/CommentedText.css
.comment {
background-color: #feeab5;
}
With all of the above code coming together, we now see text nodes with comment threads highlighted in the editor.
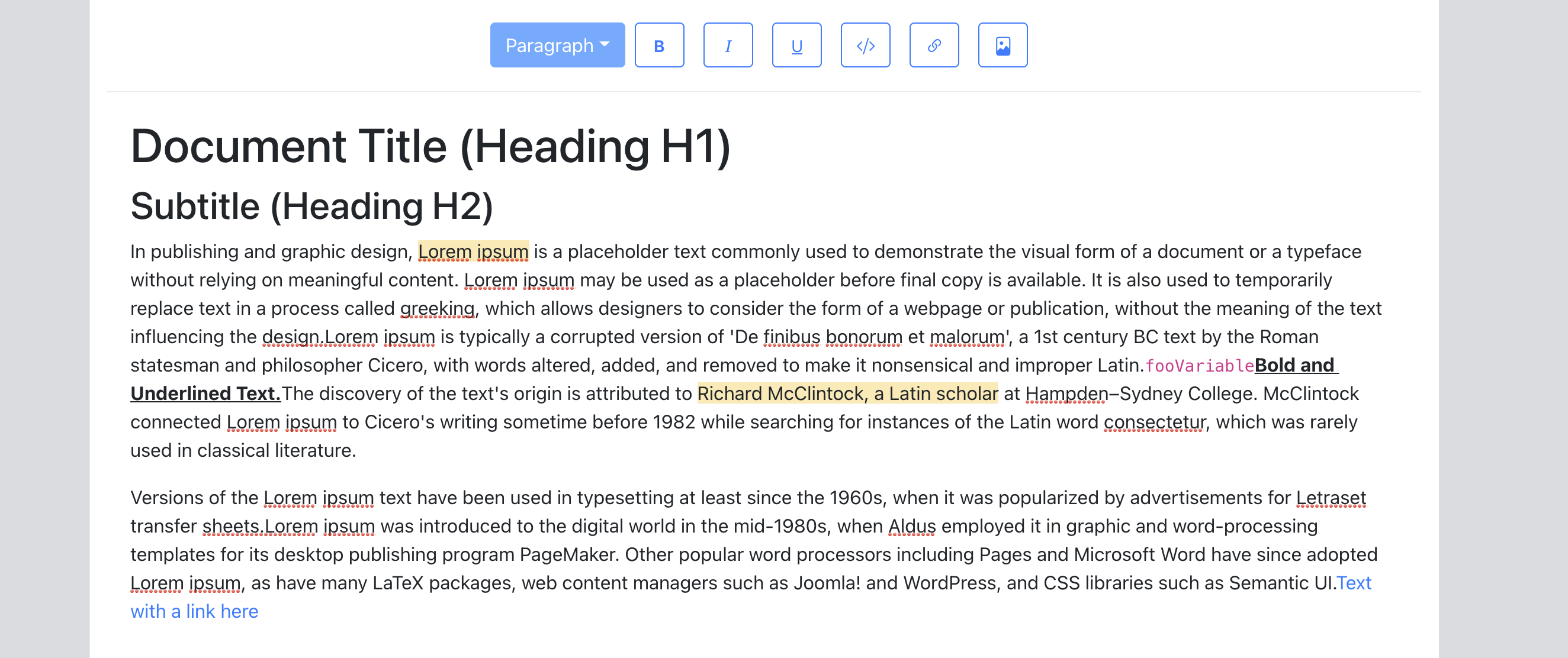
Note: The users currently cannot tell if certain text has overlapping comments on it. The entire highlighted text range looks like a single comment thread. We address that later in the article where we introduce the concept of active comment thread which lets users select a specific comment thread and be able to see its range in the editor.
UI Storage For Comments
Before we add the functionality that enables a user to insert new comments, we first setup a UI state to hold our comment threads. In this article, we use RecoilJS as our state management library to store comment threads, comments contained inside the threads and other metadata like creation time, status, comment author etc. Let’s add Recoil to our application:
> yarn add recoil
We use Recoil atoms to store these two data structures. If you’re not familiar with Recoil, atoms are what hold the application state. For different pieces of application state, you’d usually want to set up different atoms. Atom Family is a collection of atoms — it can be thought to be a Map
from a unique key identifying the atom to the atoms themselves. It’s worth going through core concepts of Recoil at this point and familiarizing ourselves with them.
For our use case, we store comment threads as an Atom family and then wrap our application in a RecoilRoot
component. RecoilRoot
is applied to provide the context in which the atom values are going to be used. We create a separate module CommentState
that holds our Recoil atom definitions as we add more atom definitions later in the article.
# src/utils/CommentState.js
import { atom, atomFamily } from "recoil";
export const commentThreadsState = atomFamily({
key: "commentThreads",
default: [],
});
export const commentThreadIDsState = atom({
key: "commentThreadIDs",
default: new Set([]),
});
Worth calling out few things about these atom definitions:
- Each atom/atom family is uniquely identified by a
key
and can be set up with a default value.
- As we build further in this article, we are going to need a way to iterate over all the comment threads which would basically mean needing a way to iterate over
commentThreadsState
atom family. At the time of writing this article, the way to do that with Recoil is to set up another atom that holds all the IDs of the atom family. We do that with commentThreadIDsState
above. Both these atoms would have to be kept in sync whenever we add/delete comment threads.
We add a RecoilRoot
wrapper in our root App
component so we can use these atoms later. Recoil’s documentation also provides a helpful Debugger component that we take as it is and drop into our editor. This component will leave console.debug
logs to our Dev console as Recoil atoms are updated in real-time.
# src/components/App.js
import { RecoilRoot } from "recoil";
export default function App() {
...
return (
<RecoilRoot>
>
...
<Editor document={document} onChange={updateDocument} />
</RecoilRoot>
);
}
# src/components/Editor.js
export default function Editor({ ... }): JSX.Element {
.....
return (
<>
<Slate>
.....
</Slate>
<DebugObserver />
</>
);
function DebugObserver(): React.Node {
// see API link above for implementation.
}
We also need to need to add code that initializes our atoms with the comment threads that already exist on the document (the ones we added to our example document in the previous section, for instance). We do that at a later point when we build the Comments Sidebar that needs to read all the comment threads in a document.
At this point, we load our application, make sure there are no errors pointing to our Recoil setup and move forward.
Adding New Comments
In this section, we add a button to the toolbar that lets the user add comments (viz. create a new comment thread) for the selected text range. When the user selects a text range and clicks on this button, we need to do the below:
- Assign a unique ID to the new comment thread being inserted.
- Add a new mark to Slate document structure with the ID so the user sees that text highlighted.
- Add the new comment thread to Recoil atoms we created in the previous section.
Let’s add a util function to EditorCommentUtils
that does #1 and #2.
# src/utils/EditorCommentUtils.js
import { Editor } from "slate";
import { v4 as uuidv4 } from "uuid";
export function insertCommentThread(editor, addCommentThreadToState) {
const threadID = uuidv4();
const newCommentThread = {
// comments as added would be appended to the thread here.
comments: [],
creationTime: new Date(),
// Newly created comment threads are OPEN. We deal with statuses
// later in the article.
status: "open",
};
addCommentThreadToState(threadID, newCommentThread);
Editor.addMark(editor, getMarkForCommentThreadID(threadID), true);
return threadID;
}
By using the concept of marks to store each comment thread as its own mark, we’re able to simply use the Editor.addMark
API to add a new comment thread on the text range selected. This call alone handles all the different cases of adding comments — some of which we described in the earlier section — partially overlapping comments, comments inside/overlapping links, comments over bold/italic text, comments spanning paragraphs and so on. This API call adjusts the node hierarchy to create as many new text nodes as needed to handle these cases.
addCommentThreadToState
is a callback function that handles step #3 — adding the new comment thread to Recoil atom . We implement that next as a custom callback hook so that it’s re-usable. This callback needs to add the new comment thread to both the atoms — commentThreadsState
and commentThreadIDsState
. To be able to do this, we use the useRecoilCallback
hook. This hook can be used to construct a callback which gets a few things that can be used to read/set atom data. The one we’re interested in right now is the set
function which can be used to update an atom value as set(atom, newValueOrUpdaterFunction)
.
# src/hooks/useAddCommentThreadToState.js
import {
commentThreadIDsState,
commentThreadsState,
} from "../utils/CommentState";
import { useRecoilCallback } from "recoil";
export default function useAddCommentThreadToState() {
return useRecoilCallback(
({ set }) => (id, threadData) => {
set(commentThreadIDsState, (ids) => new Set([...Array.from(ids), id]));
set(commentThreadsState(id), threadData);
},
[]
);
}
The first call to set
adds the new ID to the existing set of comment thread IDs and returns the new Set
(which becomes the new value of the atom).
In the second call, we get the atom for the ID from the atom family — commentThreadsState
as commentThreadsState(id)
and then set the threadData
to be its value. atomFamilyName(atomID)
is how Recoil lets us access an atom from its atom family using the unique key. Loosely speaking, we could say that if commentThreadsState
was a javascript Map, this call is basically — commentThreadsState.set(id, threadData)
.
Now that we have all this code setup to handle insertion of a new comment thread to the document and Recoil atoms, lets add a button to our toolbar and wire it up with the call to these functions.
# src/components/Toolbar.js
import { insertCommentThread } from "../utils/EditorCommentUtils";
import useAddCommentThreadToState from "../hooks/useAddCommentThreadToState";
export default function Toolbar({ selection, previousSelection }) {
const editor = useEditor();
...
const addCommentThread = useAddCommentThreadToState();
const onInsertComment = useCallback(() => {
const newCommentThreadID = insertCommentThread(editor, addCommentThread);
}, [editor, addCommentThread]);
return (
<div className="toolbar">
...
<ToolBarButton
isActive={false}
label={<i className={bi ${getIconForButton("comment")}
} />}
onMouseDown={onInsertComment}
/>
</div>
);
}
Note: We use onMouseDown
and not onClick
which would have made the editor lose focus and selection to become null
. We’ve discussed that in a little more detail in the link insertion section of the first article.
In the below example, we see the insertion in action for a simple comment thread and an overlapping comment thread with links. Notice how we get updates from Recoil Debugger confirming our state is getting updated correctly. We also verify that new text nodes are created as threads are being added to the document.
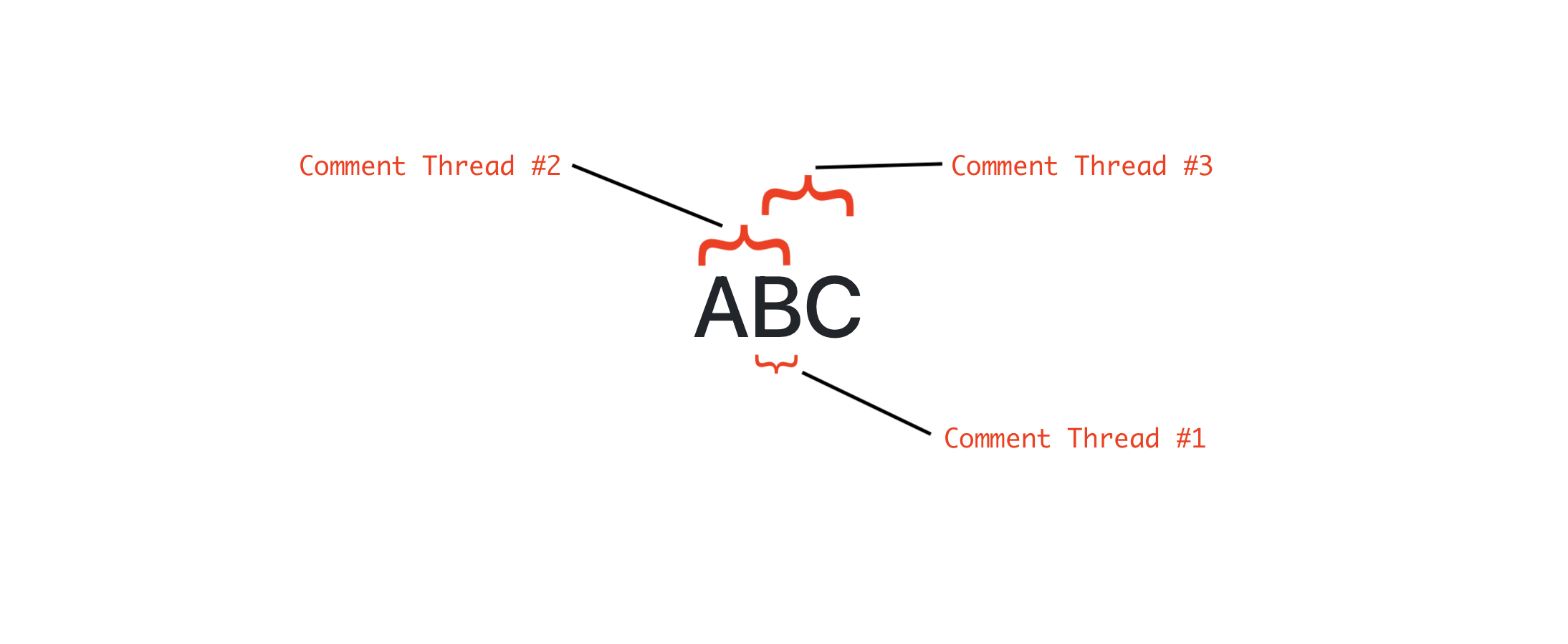
In the above example, the user inserts the following comment threads in that order:
- Comment Thread #1 over character ‘B’ (length = 1).
- Comment Thread #2 over ‘AB’ (length = 2).
- Comment Thread #3 over ‘BC’ (length = 2).
At the end of these insertions, because of the way Slate splits the text nodes with marks, we will have three text nodes — one for each character. Now, if the user clicks on ‘B’, going by the shortest length rule, we select thread #1 as it is the shortest of the three in length. If we don’t do that, we wouldn’t have a way to select Comment Thread #1 ever since it is only one-character in length and also a part of two other threads.
Although this rule makes it easy to surface shorter-length comment threads, we could run into situations where longer comment threads become inaccessible since all the characters contained in them are part of some other shorter comment thread. Let’s look at an example for that.
Let’s assume we have 100 characters (say, character ‘A’ typed 100 times that is) and the user inserts comment threads in the following order:
- Comment Thread # 1 of range 20,80
- Comment Thread # 2 of range 0,50
- Comment Thread # 3 of range 51,100
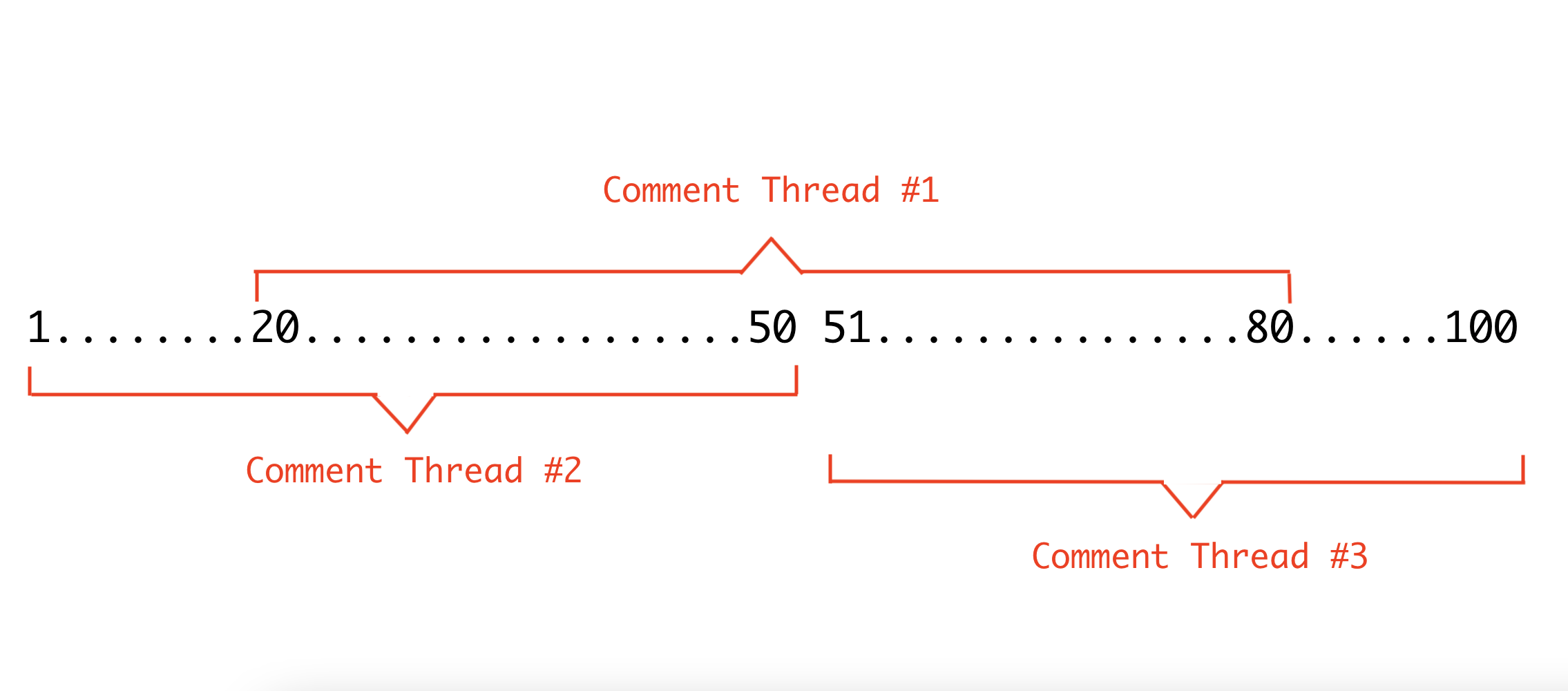
As you can see in the above example, if we follow the rule we just described here, clicking on any character between #20 and #80, would always select threads #2 or #3 since they are shorter than #1 and hence #1 would not be selectable. Another scenario where this rule can leave us undecided as to which comment thread to select is when there are more than one comment threads of the same shortest length on a text node.
For such combination of overlapping comments and many other such combinations that one could think of where following this rule makes a certain comment thread inaccessible by clicking on text, we build a Comments Sidebar later in this article which gives user a view of all the comment threads present in the document so they can click on those threads in the sidebar and activate them in the editor to see the range of the comment. We still would want to have this rule and implement it as it should cover a lot of overlap scenarios except for the less-likely examples we cited above. We put in all this effort around this rule primarily because seeing highlighted text in the editor and clicking on it to comment is a more intuitive way of accessing a comment on text than merely using a list of comments in the sidebar.
Insertion Rule
The rule is:
“If the text user has selected and is trying to comment on is already fully covered by comment thread(s), don’t allow that insertion.”
This is so because if we did allow this insertion, each character in that range would end up having at least two comment threads (one existing and another the new one we just allowed) making it difficult for us to determine which one to select when the user clicks on that character later.
Looking at this rule, one might wonder why we need it in the first place if we already have the Shortest Comment Range Rule that allows us to select the smallest text range. Why not allow all combinations of overlaps if we can use the first rule to deduce the right comment thread to show? As some of the examples we’ve discussed earlier, the first rule works for a lot of scenarios but not all of them. With the Insertion Rule, we try to minimize the number of scenarios where the first rule cannot help us and we have to fallback on the Sidebar as the only way for the user to access that comment thread. Insertion Rule also prevents exact-overlaps of comment threads. This rule is commonly implemented by a lot of popular editors.
Below is an example where if this rule didn’t exist, we would allow the Comment Thread #3 and then as a result of the first rule, #3 would not be accessible since it would become the longest in length.
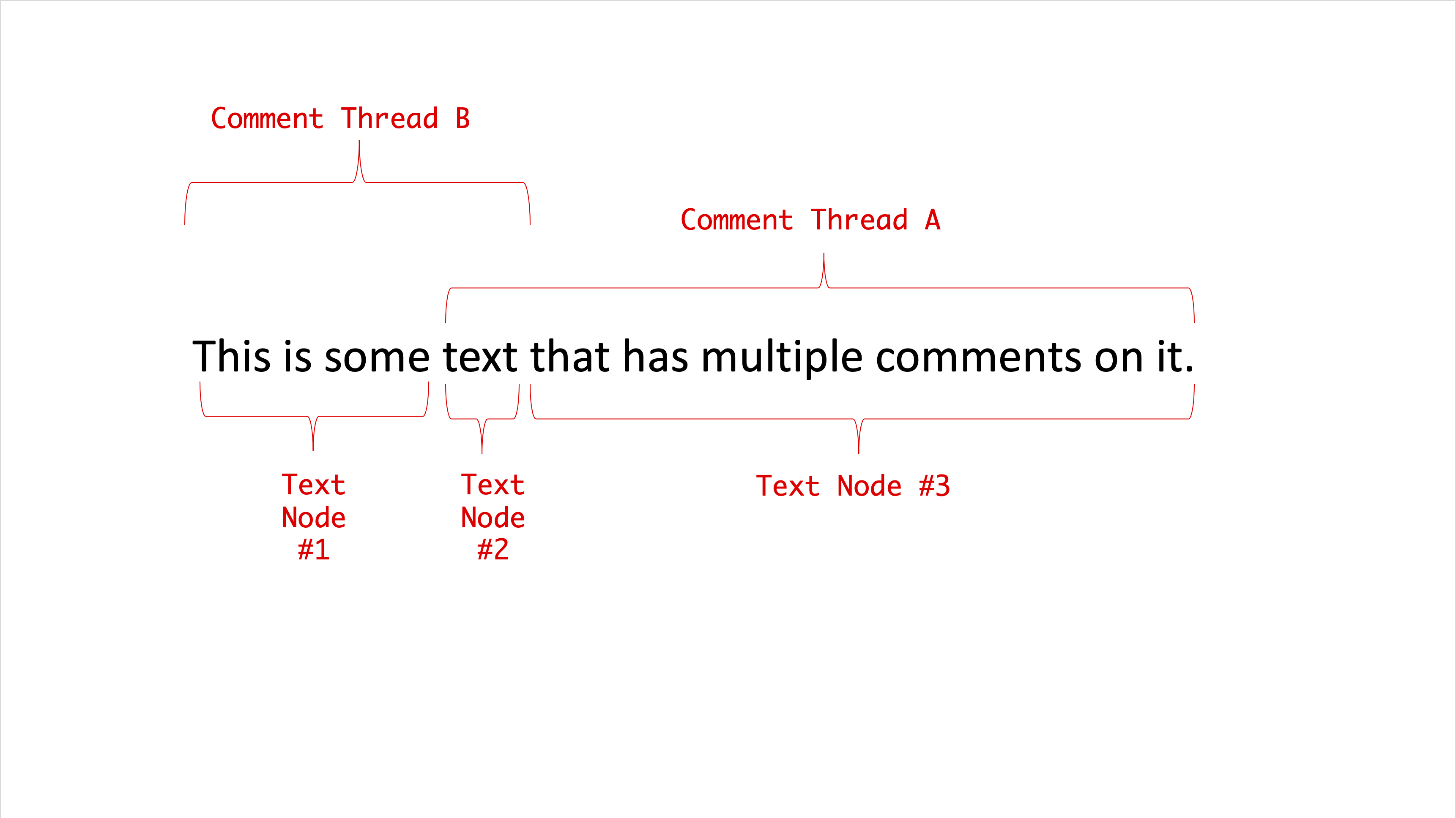
In this example, let’s assume we don’t wait for intersection to become 0 and just stop when we reach the edge of a comment thread. Now, if the user clicked on #2 and we start traversal in reverse direction, we’d stop at the start of text node #2 itself since that’s the start of the comment thread A. As a result, we might not compute the comment thread lengths correctly for A & B. With the implementation above traversing the farthest edges (text nodes 1,2, and 3), we should get B as the shortest comment thread as expected.
To see the implementation visually, below is a walkthrough with a slideshow of the iterations. We have two comment threads A and B that overlap each other over text node #3 and the user clicks on the overlapping text node #3.
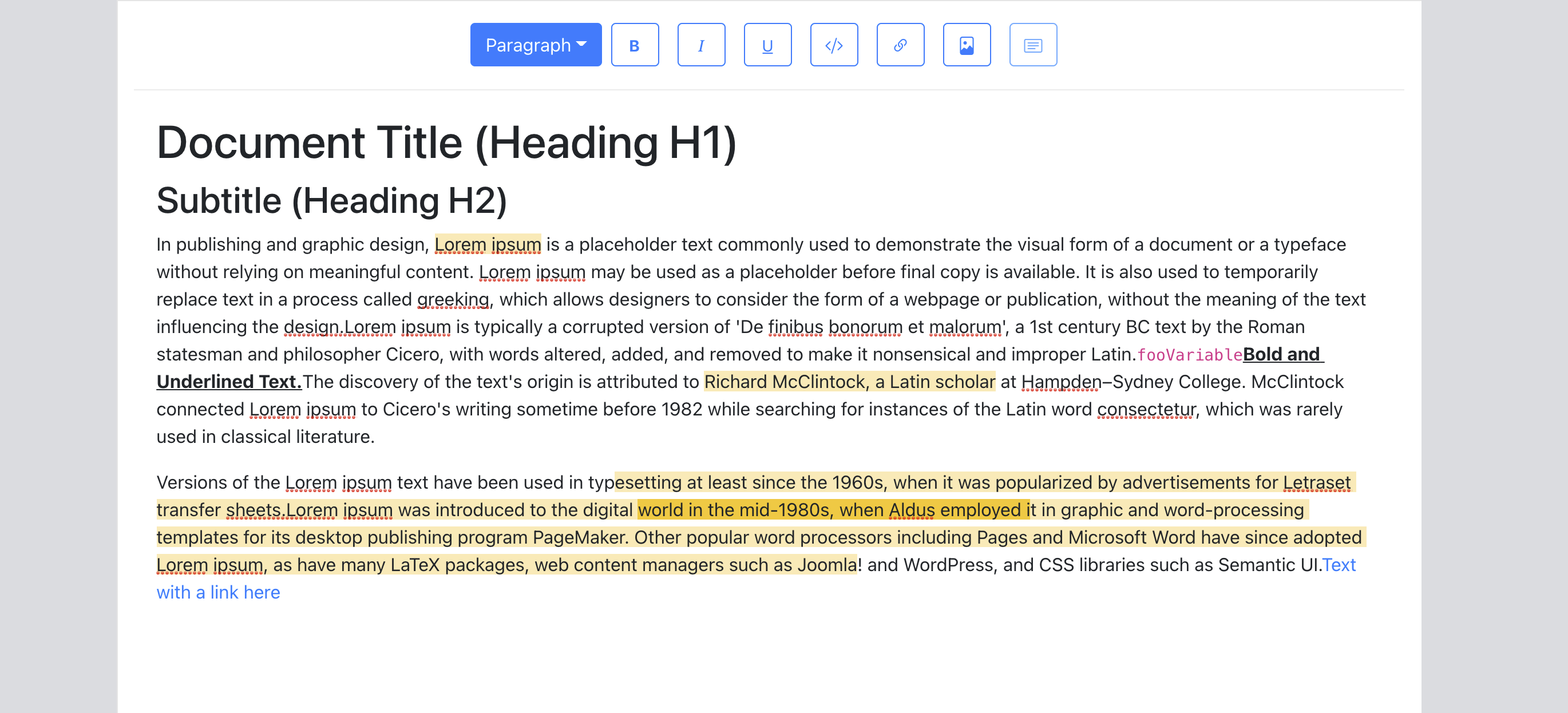
Now that we have all the code in to make selection of comment threads work, let’s see it in action. To test our traversal code well, we test some straightforward cases of overlap and some edge cases like:
- Clicking on a commented text node at the start/end of the editor.
- Clicking on a commented text node with comment threads spanning multiple paragraphs.
- Clicking on a commented text node right before an image node.
- Clicking on a commented text node overlapping links.
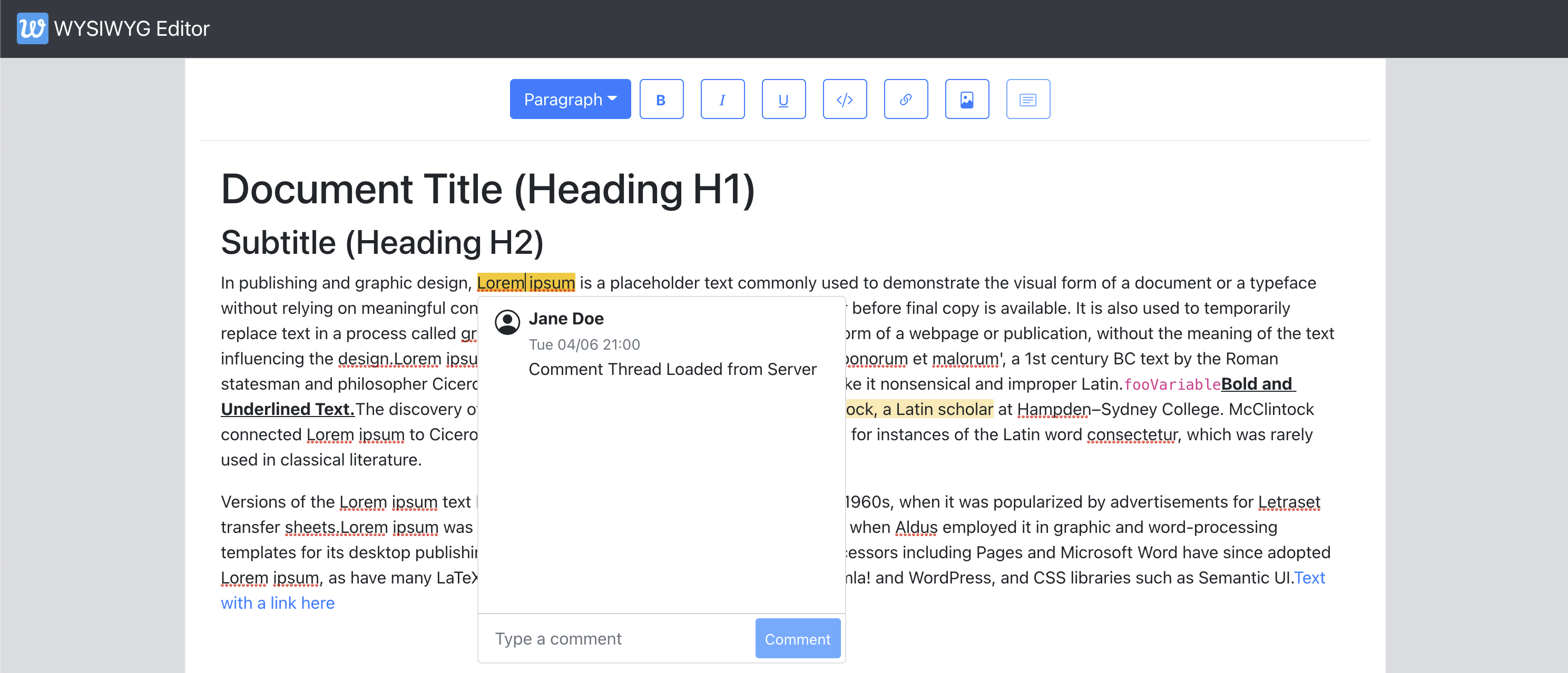
Now that our state is correctly initialized, we can start implementing the sidebar. All our comment threads in the UI are stored in the Recoil atom family — commentThreadsState
. As highlighted earlier, the way we iterate through all the items in a Recoil atom family is by tracking the atom keys/ids in another atom. We’ve been doing that with commentThreadIDsState
. Let’s add the CommentSidebar
component that iterates through the set of ids in this atom and renders a CommentThread
component for each.
# src/components/CommentsSidebar.js
import "./CommentSidebar.css";
import {commentThreadIDsState,} from "../utils/CommentState";
import { useRecoilValue } from "recoil";
export default function CommentsSidebar(params) {
const allCommentThreadIDs = useRecoilValue(commentThreadIDsState);
return (
<Card className={"comments-sidebar"}>
<Card.Header>Comments</Card.Header>
<Card.Body>
{Array.from(allCommentThreadIDs).map((id) => (
<Row key={id}>
<Col>
<CommentThread id={id} />
</Col>
</Row>
))}
</Card.Body>
</Card>
);
}
Now, we implement the CommentThread
component that listens to the Recoil atom in the family corresponding to the comment thread it is rendering. This way, as the user adds more comments on the thread in the editor or changes any other metadata, we can update the sidebar to reflect that.
As the sidebar could grow to be really big for a document with a lot of comments, we hide all comments but the first one when we render the sidebar. The user can use the ‘Show/Hide Replies’ button to show/hide the entire thread of comments.
# src/components/CommentSidebar.js
function CommentThread({ id }) {
const { comments } = useRecoilValue(commentThreadsState(id));
const [shouldShowReplies, setShouldShowReplies] = useState(false);
const onBtnClick = useCallback(() => {
setShouldShowReplies(!shouldShowReplies);
}, [shouldShowReplies, setShouldShowReplies]);
if (comments.length === 0) {
return null;
}
const [firstComment, ...otherComments] = comments;
return (
<Card
body={true}
className={classNames({
"comment-thread-container": true,
})}
>
<CommentRow comment={firstComment} showConnector={false} />
{shouldShowReplies
? otherComments.map((comment, index) => (
<CommentRow key={comment-${index}
} comment={comment} showConnector={true} />
))
: null}
{comments.length > 1 ? (
<Button
className={"show-replies-btn"}
size="sm"
variant="outline-primary"
onClick={onBtnClick}
>
{shouldShowReplies ? "Hide Replies" : "Show Replies"}
</Button>
) : null}
</Card>
);
}
We’ve reused the CommentRow
component from the popover although we added a design treatment using showConnector
prop that basically makes all the comments look connected with a thread in the sidebar.
Now, we render the CommentSidebar
in the Editor
and verify that it shows all the threads we have in the document and correctly updates as we add new threads or new comments to existing threads.
# src/components/Editor.js
return (
<>
<Slate ... >
.....
<div className={"sidebar-wrapper"}>
<CommentsSidebar />
</div>
</Slate>
</>
);
We now move on to implementing a popular Comments Sidebar interaction found in editors:
Clicking on a comment thread in the sidebar should select/activate that comment thread. We also add a differential design treatment to highlight a comment thread in the sidebar if it’s active in the editor. To be able to do so, we use the Recoil atom — activeCommentThreadIDAtom
. Let’s update the CommentThread
component to support this.
# src/components/CommentsSidebar.js
function CommentThread({ id }) {
const [activeCommentThreadID, setActiveCommentThreadID] = useRecoilState(
activeCommentThreadIDAtom
);
const onClick = useCallback(() => {
setActiveCommentThreadID(id);
}, [id, setActiveCommentThreadID]);
...
return (
<Card
body={true}
className={classNames({
"comment-thread-container": true,
"is-active": activeCommentThreadID === id,
})}
onClick={onClick}
>
....
</Card>
);
If we look closely, we have a bug in our implementation of sync-ing the active comment thread with the sidebar. As we click on different comment threads in the sidebar, the correct comment thread is indeed highlighted in the editor. However, the Comment Popover doesn’t actually move to the changed active comment thread. It stays where it was first rendered. If we look at the implementation of the Comment Popover, it renders itself against the first text node in the editor’s selection. At that point in the implementation, the only way to select a comment thread was to click on a text node so we could conveniently rely on the editor’s selection since it was updated by Slate as a result of the click event. In the above onClick
event, we don’t update the selection but merely update the Recoil atom value causing Slate’s selection to remain unchanged and hence the Comment Popover doesn’t move.
A solution to this problem is to update the editor’s selection along with updating the Recoil atom when the user clicks on the comment thread in the sidebar. The steps do this are:
- Find all text nodes that have this comment thread on them that we are going to set as the new active thread.
- Sort these text nodes in the order in which they appear in the document (We use Slate’s
Path.compare
API for this).
- Compute a selection range that spans from the start of the first text node to the end of the last text node.
- Set the selection range to be the editor’s new selection (using Slate’s
Transforms.select
API).
If we just wanted to fix the bug, we could just find the first text node in Step #1 that has the comment thread and set that to be the editor’s selection. However, it feels like a cleaner approach to select the entire comment range as we really are selecting the comment thread.
Let’s update the onClick
callback implementation to include the steps above.
const onClick = useCallback(() => {
const textNodesWithThread = Editor.nodes(editor, {
at: [],
mode: "lowest",
match: (n) => Text.isText(n) && getCommentThreadsOnTextNode(n).has(id),
});
let textNodeEntry = textNodesWithThread.next().value;
const allTextNodePaths = [];
while (textNodeEntry != null) {
allTextNodePaths.push(textNodeEntry[1]);
textNodeEntry = textNodesWithThread.next().value;
}
// sort the text nodes
allTextNodePaths.sort((p1, p2) => Path.compare(p1, p2));
// set the selection on the editor
Transforms.select(editor, {
anchor: Editor.point(editor, allTextNodePaths[0], { edge: "start" }),
focus: Editor.point(
editor,
allTextNodePaths[allTextNodePaths.length - 1],
{ edge: "end" }
),
});
// Update the Recoil atom value.
setActiveCommentThreadID(id);
}, [editor, id, setActiveCommentThreadID]);
Note: allTextNodePaths
contains the path to all the text nodes. We use the Editor.point
API to get the start and end points at that path. The first article goes through Slate’s Location concepts. They’re also well-documented on Slate’s documentation.
Let’s verify that this implementation does fix the bug and the Comment Popover moves to the active comment thread correctly. This time, we also test with a case of overlapping threads to make sure it doesn’t break there.
With the bug fix, we’ve enabled another sidebar interaction that we haven’t discussed yet. If we have a really long document and the user clicks on a comment thread in the sidebar that’s outside the viewport, we’d want to scroll to that part of the document so the user can focus on the comment thread in the editor. By setting the selection above using Slate’s API, we get that for free. Let’s see it in action below.
With that, we wrap our implementation of the sidebar. Towards the end of the article, we list out some nice feature additions and enhancements we can do to the Comments Sidebar that help elevate the Commenting and Review experience on the editor.
Resolving And Re-Opening Comments
In this section, we focus on enabling users to mark comment threads as ‘Resolved’ or be able to re-open them for discussion if needed. From an implementation detail perspective, this is the status
metadata on a comment thread that we change as the user performs this action. From a user’s perspective, this is a very useful feature as it gives them a way to affirm that the discussion about something on the document has concluded or needs to be re-opened because there are some updates/new perspectives, and so on.
To enable toggling the status, we add a button to the CommentPopover
that allows the user to toggle between the two statuses: open
and resolved
.
# src/components/CommentThreadPopover.js
export default function CommentThreadPopover({
editorOffsets,
selection,
threadID,
}) {
…
const [threadData, setCommentThreadData] = useRecoilState(
commentThreadsState(threadID)
);
...
const onToggleStatus = useCallback(() => {
const currentStatus = threadData.status;
setCommentThreadData((threadData) => ({
...threadData,
status: currentStatus === "open" ? "resolved" : "open",
}));
}, [setCommentThreadData, threadData.status]);
return (
<NodePopover
...
header={
<Header
status={threadData.status}
shouldAllowStatusChange={threadData.comments.length > 0}
onToggleStatus={onToggleStatus}
/>
}
>
<div className={"comment-list"}>
...
</div>
</NodePopover>
);
}
function Header({ onToggleStatus, shouldAllowStatusChange, status }) {
return (
<div className={"comment-thread-popover-header"}>
{shouldAllowStatusChange && status != null ? (
<Button size="sm" variant="primary" onClick={onToggleStatus}>
{status === "open" ? "Resolve" : "Re-Open"}
</Button>
) : null}
</div>
);
}
Before we test this, let’s also give the Comments Sidebar a differential design treatment for resolved comments so that the user can easily detect which comment threads are un-resolved or open and focus on those if they want to.
# src/components/CommentsSidebar.js
function CommentThread({ id }) {
...
const { comments, status } = useRecoilValue(commentThreadsState(id));
...
return (
<Card
body={true}
className={classNames({
"comment-thread-container": true,
"is-resolved": status === "resolved",
"is-active": activeCommentThreadID === id,
})}
onClick={onClick}
>
...
</Card>
);
}
Conclusion
In this article, we built the core UI infrastructure for a Commenting System on a Rich Text Editor. The set of functionalities we add here act as a foundation to build a richer Collaboration Experience on an editor where collaborators could annotate parts of the document and have conversations about them. Adding a Comments Sidebar gives us a space to have more conversational or review-based functionalities to be enabled on the product.
Along those lines, here are some of features that a Rich Text Editor could consider adding on top of what we built in this article:
- Support for
@
mentions so collaborators could tag one another in comments;
- Support for media types like images and videos to be added to comment threads;
- Suggestion Mode at the document level that allows reviewers to make edits to the document that appear as suggestions for changes. One could refer to this feature in Google Docs or Change Tracking in Microsoft Word as examples;
- Enhancements to the sidebar to search conversations by keyword, filter threads by status or comment author(s), and so on.